- SUN: Groovy Support: WebSynergy and the associated Portal Pack 3.0 continue to add features as part of its partnership with Liferay. Besides Ruby and PHP, you can now run Groovy apps as JSR-286 portlets deployed to WebSynergy as described by Frerk Meyer.
- Oracle (BEA): no news.
- IBM: Finally, WebSphere Application Server 7 is out. This means that IBM has joined the Java EE 5 vendor parade. With this release, the server also supports JSR-286 portlets as described in a very detailed release post.
- eXo: The next release of eXo Portal (v2.5) will support right-to-left (RTL) languages (see picture) like Arab or Hebrew. A wiki dedicated to this RTL framework provides more details.
- JBoss:
> JSR-168 Portal: Release of JBoss Portal 2.6.7, a maintenance release of JBoss Portal 2.6: Main improvements have been done in scalability. JBoss claims that you can tune your portal application on a 1-node environment as the new version scales linearly.
> JSR-286 Portal: Release 2.7 is in the process to go GA.
- Eclipse: The first milestone of Eclipse Portal Pack 2.0 has been released. The portal pack consists of three plugins: JSR-286/168 Portlet Wizard, WebSynergy Plugin and the OpenPortal Plugin.
- NetBeans and IntelliJIDEA: no news regarding portal support.
Wednesday, October 29, 2008
Portal Update October 2008
Friday, October 24, 2008
Giving EJB 3.1 a Test Drive
- Singleton Beans
- Simplified EJB packaging
- Simplified EJB without Interfaces
- Unified JNDI naming
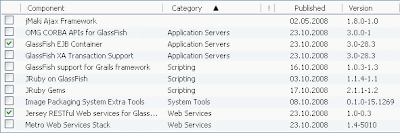
Beside the EJB Container we'll also install the new JAX-RS REST API to access the EJB. The JAX-RS JSR has just recently gone final, and is definitely also worth a look. Once the installation is done, we'll switch to the IDE to code our beans. I'm using Eclipse with the Maven 2 plugin to get started quickly.
Choose New Maven Project and select the maven-archetype-webapp archetype as shown in the screenshot below. We're naming our project "ejbtestdrive" - note the name as it will be used a couple of times in the further process. Finish the create wizard and add the src/main/java folder as a source folder in Eclipse after creating it.

To resolve our dependencies, lets first configure the Maven repositories to download the EJB 3.1 as well as the JAX-RS API. Add the following lines to your pom.xml:
<repositories>
<repository>
<id>glassfish</id>
<name>Glassfish Repository</name>
<url>http://download.java.net/maven/glassfish</url>
</repository>
<repository>
<id>java.net</id>
<name>java.net Repository</name>
<url>http://download.java.net/maven/2</url>
</repository>
</repositories>
Which allows us to download following dependencies:
<dependencies>
<dependency>
<groupId>javax.ejb</groupId>
<artifactId>ejb-api-3.1-alpha</artifactId>
<version>10.0-SNAPSHOT</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-server</artifactId>
<version>1.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
Almost done with the Maven setup. We'll just add the GlassFish Maven plugin to our build and set the compiler to deal with Java 6 (Java 5 should be fine too):
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>1.6</source>
<target>1.6</target>
</configuration>
</plugin>
<plugin>
<groupId>org.glassfish.maven.plugin</groupId>
<artifactId>maven-glassfish-plugin</artifactId>
<version>2.1</version>
<configuration>
<glassfishDirectory>${glassfish.home}</glassfishDirectory>
<domain>
<name>domain1</name>
<adminPort>4848</adminPort>
</domain>
<user>${domain.username}</user>
<adminPassword>${domain.password}</adminPassword>
<components>
<component>
<name>${project.artifactId}</name>
<artifact>${project.build.directory}/${project.build.finalName}.${project.packaging}</artifact>
</component>
</components>
<autoCreate>true</autoCreate>
<debug>true</debug>
<echo>true</echo>
</configuration>
</plugin>
</plugins>
</build>
The referenced properties should be defined in a Maven profile, but for simplicity we'll just add them now to our POM (just replace the values with your installation location and your username/password):
<properties>
<glassfish.home>E:\work\servers\glassfish\glassfishv3-prelude\glassfish</glassfish.home>
<domain.username>admin</domain.username>
<domain.password>adminadmin</domain.password>
</properties>
You're now ready to run the WAR file on GlassFish. Just run
mvn package glassfish:deploy
to start GlassFish and get your WAR file deployed (Note: If the Maven plugin complains about asadmin not be a Win32 application, just remove the UNIX script so the .bat will be taken).
Finally, time to write some code! Go back to Eclipse and create a package. Our EJB is going to be a (surprise surprise) HelloWorld bean. Create a new class which looks like the following:
package com.ctp.ejb;In case Eclipse complains about the compiler compliance, just set the Java project facet in the project's properties to something > 1.4. You've now created one of the new Singleton EJBs. The bean is going to remember everybody who said "hello" and give back a friendly greeting. Note that there is no local or remote interface at all! Also using a global state in a bean is something nasty to achieve before EJB 3.1. Run
import java.util.LinkedHashSet;
import java.util.Set;
import javax.ejb.Singleton;
/**
* The friendly first EJB 3.1 bean.
* @author [you!]
*/
@Singleton
public class HelloWorldBean {
private Set<String> names = new LinkedHashSet<String>();
public String hello(String name) {
names.add(name);
return "Hello " + names;
}
}
mvn package glassfish:redeploy
to deploy your bean - noteably in just a simple WAR file! Did you notice how fast this deploys?
Now it's time to access our bean over JAX-RS. Create another class which looks like this:
package com.ctp.ejb;
import javax.naming.InitialContext;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
/**
* A RESTful bean being hopefully quite busy!
* @author [you!]
*/
@Path("/hello/{username}")
public class HelloWorld {
@GET
@Produces("text/plain")
public String hello(@PathParam("username") String name) throws Exception {
HelloWorldBean hello = (HelloWorldBean)
new InitialContext().lookup("java:global/ejbtestdrive/HelloWorldBean");
return hello.hello(name);
}
}
Simple enough, right? This is taking requests under /hello/... with a username, looking up the bean in JNDI and call it's hello method. Did you notice the format of the JNDI lookup? Yes, this is finally standardized, making your applications much more portable! In case you named your application differently, make sure the names match between global/ and /HelloWorldBean.
Further details on the JAX-RS functionalities can be found here.
As a last step, we need to add the Jersey servlet to our web.xml. Modify it to match this snippet:
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
version="2.5">
<display-name>embeddable</display-name>
<servlet>
<servlet-name>Jersey Web Application</servlet-name>
<servlet-class>com.sun.jersey.spi.container.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>com.sun.jersey.config.property.packages</param-name>
<param-value>com.ctp</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>Jersey Web Application</servlet-name>
<url-pattern>/*</url-pattern>
</servlet-mapping>
</web-app>
Check that your package name matches in the Servlets init param. Now package and redeploy - and we're ready to go! Just go to http://localhost:8080/ejbtestdrive/hello/[your name] to see you greeted by your new EJB! Experiment with different names and browser sessions and see the global state preserved.
Hope you enjoyed this little test drive. Let us know on how you're using EJB 3.1 and what your experience is with it!
Sunday, October 19, 2008
Java People Spotlight: Piyush Shah
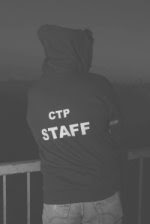
Java Community Role:
Developer [aka 0800-DEBUG: "Who you gonna call?" - "Bug-Shah Buster!!!!"]
My Master Kung-Fu Skills:
Apache Tomcat 6, WebLogic 10, Javascript
I'd be excited to get my hands dirty on:
Spring and JBoss Seam
Q&A
Q: Hi Piyush, how would your message look like if you would have to tell it via Twitter what you are currently doing?
A: Trying to break and rebuild Interwoven Teamsite to incorporate Xopus.
Q: What was the greates piece of code you have ever written so far?
A: request.getRequestDispatcher(URL).forward(request, response);
A: "Developing an application is like making a movie"
Q: What is the best quote you have heard from our managers?
A: "XYZ technology, you can do it. It’s a piece of cake."
Q: What is the most cutting-edge technology or framework you actually used on projects?
A: Porting my custom code using SSH client on Solaris server and making sure it is Java1.4 compatible.
Q: What is your favorite podcast?
A: TSS (TheServerSide.com)
Q: Which Java book can you recommend?
A: SCJP6 - by Katherine Sierra
Tuesday, October 7, 2008
Java People Spotlight: Douglas Rodrigues
Java Community Role:
Developer [aka Java Code Spitter]
My Master Kung-Fu Skills:
Write code that (almost) anyone can read and understand in the future.
I'd be excited to get my hands dirty on:
JBoss DNA and IntelliJ IDEA 8.
Q&A
Q: Hi Douglas, how would your message look like if you would have to tell it via Twitter what you are currently doing?
A: while (!weekend) { iTunes.open(); while (!night) { iTunes.play(); doCode(); iTunes.pause(); getCoffee(); } iTunes = null; } // One-liners rocks! \o/
Q': and where is the exception handling??!!! ;-)
Q: What was the greates piece of code you have ever written so far?
A: An image to HTML converter. It takes a 30kB image and produces a 5MB HTML file.
A: "The nice thing about standards is that there are so many of them to choose from", by Andrew S. Tanenbaum.
Q: What is the best quote you have heard from our managers?
A: Well, I hear "we're all in sales" at least once every month.
Q: What is the most cutting-edge technology or framework you actually used on projects?
A: Java Content Repository API (JCR).
Q: What is your favorite podcast?
A: I'm not a big fan of technology related podcasts, but I tried Java Posse and it's kinda funny.
Q: Which Java book can you recommend and for what reason?
A: Head First Design Patterns